Securing access to data in Azure Cosmos DB
Microsoft DP-200 exam is getting retired on June 30, 2021. A new replacement exam Data Engineering on Microsoft Azure (DP-203) is available.
In this tutorial, we will learn and understand about securing access to data in Microsoft Azure Cosmos DB.
Azure Cosmos DB uses two types of keys for authenticating users and providing access to its data and resources:
- Master Keys
- Resource Tokens
Master keys
This keys is for providing access to all the administrative resources for the database account. The Master keys:
- Firstly, provide access to accounts, databases, users, and permissions.
- Secondly, cannot be used to provide granular access to containers and documents.
- Thirdly, are created during the creation of an account.
- Lastly, can be regenerated at any time.
However, each account consists of two Master keys that are a primary key and secondary key. And, the purpose of dual keys is so that you can regenerate, or roll keys, providing continuous access to your account and data.
In addition, for the two master keys for the Cosmos DB account, there are two read-only keys. Where these read-only keys only allow read operations on the account. Where read-only keys do not provide any access for reading permissions resources.
Key rotation
The process of rotating your master key is simple.
- Firstly, navigate to the Azure portal to retrieve your secondary key.
- Secondly, replace your primary key with your secondary key in your application. Then, make sure that all the Cosmos DB clients across all the deployments are promptly restarted and will start using the updated key.
- Thirdly, rotate the primary key in the Azure portal.
- After that, validating the new primary key works against all resources. However, the key rotation process can take anywhere from less than a minute to hours depending on the size of the Cosmos DB account.
- Lastly, replace the secondary key with the new primary key.
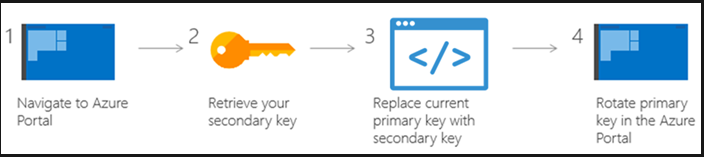
Code sample for using a master key
The code sample below illustrates how to use a Cosmos DB account endpoint and master key to instantiate a DocumentClient and create a database:
C#
//Read the Azure Cosmos DB endpointUrl and authorization keys from config.
//These values are available from the Azure portal on the Azure Cosmos DB account blade under “Keys”.
//Keep these values in a safe and secure location. Together they provide Administrative access for your Azure Cosmos DB account.
private static readonly string endpointUrl = ConfigurationManager.AppSettings[“EndPointUrl”];
private static readonly string authorizationKey = ConfigurationManager.AppSettings[“AuthorizationKey”];
CosmosClient client = new CosmosClient(endpointUrl, authorizationKey);
Resource tokens
Resource tokens is for providing access to the application resources within a database. They:
- Firstly, provides access to specific containers, partition keys, documents, attachments, stored procedures, triggers, and UDFs.
- Secondly, are created when a user is granted permissions to a specific resource.
- Thirdly, are recreated when a permission resource is acted upon by POST, GET, or PUT call.
- Fourthly, use a hash resource token specifically constructed for the user, resource, and permission.
- Fifthly, are time bound with a customizable validity period. However, the default valid time span is one hour. And, token lifetime, however, may be explicitly specified, up to a maximum of five hours.
- Next, provide a safe alternative to giving out the master key.
- Lastly, enable clients to read, write, and delete resources in the Cosmos DB account according to the permissions they’ve been granted.
However, you can use a resource token when you want to provide access to resources in your Cosmos DB account to a client that cannot be trusted with the master key.
Cosmos DB resource tokens offers a secure alternative for enabling clients to read, write, and delete resources in your Cosmos DB account. This is according to the permissions you’ve granted and without need for either a master or read only key.
Next, there is a typical design pattern whereby resource tokens may be requested, generated, and delivered to clients:
- Firstly, a mid-tier service is set up to serve a mobile application to share user photos.
- Secondly, the mid-tier service possesses the master key of the Cosmos DB account.
- Thirdly, the photo app is installed on end-user mobile devices.
- Then, on login, the photo app establishes the identity of the user with the mid-tier service. However, this mechanism of identity establishment is totally up to the application.
- After establishing the identity, the mid-tier service requests permissions based on the identity.
- Next, the mid-tier service sends a resource token back to the phone app.
- Then, the phone app can continue to use the resource token to directly access Cosmos DB resources with given permissions by the resource token and for the interval allowed by the resource token.
- Lastly, when the resource token expires, subsequent requests receive a 401 unauthorized exception. And, at this point, the phone app re-establishes the identity and requests for a new resource token.
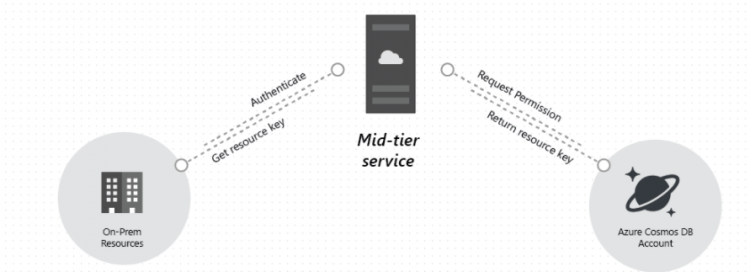
Users
Azure Cosmos DB users have links with a Cosmos database. Where each database can consist of zero or more Cosmos DB users. The code sample below shows how to create a Cosmos DB user using the Azure Cosmos DB .NET SDK v3.
C#
//Create a user.
Database database = benchmark.client.GetDatabase(“SalesDatabase”);
User user = await database.CreateUserAsync(“User 1”);
Permissions
A permission resource has links with a user and assigned at the container as well as partition key level. In this, each user may contain zero or more permissions. Moreover, a permission resource provides access to a security token that the user needs when trying to access a specific container or data in a specific partition key. However, there are two available access levels that permission resource provide:
- Firstly, All in which the user has full permission on the resource.
- Secondly, Read in which the user can only read the contents of the resource. But, they cannot perform write, update, or delete operations on the resource.
Code sample for creating permission
The code sample below shows how to create a permission resource, read the resource token of the permission resource, and associate the permissions with the user created above.
C#
// Creating a permission on a container and specific partition key value
Container container = client.GetContainer(“SalesDatabase”, “OrdersContainer”);
user.CreatePermissionAsync(
new PermissionProperties(
id: “permissionUser1Orders”,
permissionMode: PermissionMode.All,
container: benchmark.container,
resourcePartitionKey: new PartitionKey(“012345”)));
Code sample for reading permission for user
The code snippet below shows how to retrieve the permission associated with the user created above. And it instantiates a new CosmosClient on behalf of the user, scoped to a single partition key.
C#
//Read a permission, create user client session.
PermissionProperties permissionProperties = await user.GetPermission(“permissionUser1Orders”)
CosmosClient client = new CosmosClient(accountEndpoint: “MyEndpoint”, authKeyOrResourceToken: permissionProperties.Token);
Adding users and assigning roles
For adding Azure Cosmos DB account reader access to your user account, then have a subscription owner perform the following steps in the Azure portal.
- Firstly, open the Azure portal, and select your Azure Cosmos DB account.
- Secondly, click the Access control (IAM) tab, and then click + Add role assignment.
- Thirdly, in the Add role assignment pane, in the Role box, select Cosmos DB Account Reader Role.
- Then, in the Assign access to box, select Azure AD user, group, or application.
- After that, select the user, group, or application in your directory to which you wish to grant access. However, you can search the directory by display name, email address, or object identifiers. Then, the selected user, group, or application appears in the selected members list.
- Lastly, click Save.
Deleting or exporting user data
Azure Cosmos DB enables you to search, select, modify and delete any personal data in the database or collections. Moreover, it provides APIs to find and delete personal data and it’s your responsibility to use the APIs and define logic required to erase the personal data. Further, each multi-model API (SQL, MongoDB, Gremlin, Cassandra, Table) provides different language SDKs that contain methods for searching and deleting personal data. And, you can also enable the time to live (TTL) feature for deleting data automatically after a specified period, without incurring any additional cost.
Reference: Microsoft Documentation